Email Verification API
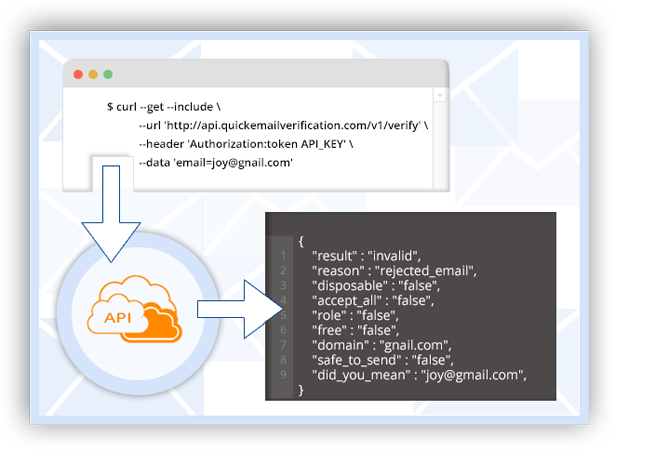
Collect better. Connect better. Integrate our API in webforms, marketing platforms or anywhere to collect high-quality, deliverable email addresses.
Learn more »Get more. Do more. Our email verification API gives you all the options you need. Choose libraries from PHP, Node.js, Ruby, Python, and more!
Learn more »Automate easily. Deliver quickly. Use the API to automate email list cleaning process and spend more time getting business and serving customers.
Learn more »Real time email verification lets you verify email addresses as they are typed in the signup form. Our REST API instantly checks if the address is valid and helps customers correct email typos. It also prevents disposable, invalid or shared addresses from getting into your list and lowering your mailing list quality.
Easily turn the email validation process into a highly efficient automation within your platform. No matter how big your list size, our high-quality bulk email verifier will remove all hard bounces and any toxic emails from your list.
Just copy-paste a few lines of code to integrate email verification API within your platform.
Choose from our ready to use API libraries for quick and easy integration.
This API endpoint is ideal for verifying single email at its entry level. You can verify an email to check its validity before allowing it to enter your email database.
curl --get --include \ --url "https://api.quickemailverification.com/v1/verify?email=EMAIL&apikey=API_KEY"
<?php require_once 'vendor/autoload.php'; // Replace API_KEY with your API Key $client = new QuickEmailVerification\Client('API_KEY'); $quickemailverification = $client->quickemailverification(); // Email address which need to be verified $response = $quickemailverification->verify("EMAIL"); ?>
var QuickEmailVerification = require('quickemailverification'); // Replace API_KEY with your API Key var client = QuickEmailVerification.client('API_KEY').quickemailverification(); // Email address which need to be verified client.verify("EMAIL", function (err, response) { // Print response object console.log(response.body); });
import quickemailverification # Replace API_KEY with your API Key client = quickemailverification.Client('API_KEY') quickemailverification = client.quickemailverification() # Email address which need to be verified response = quickemailverification.verify('EMAIL') print(response.body) # The response is in the body attribute
require "quickemailverification" # Replace API_KEY with your API Key client = QuickEmailVerification::Client.new('API_KEY') quickemailverification = client.quickemailverification() # Email address which need to be verified response = quickemailverification.verify("EMAIL") puts response.body # The response is in the body attribute
import "github.com/quickemailverification/quickemailverification-go" // Replace API_KEY with your API Key qev := quickemailverification.CreateClient('API_KEY') // Email address which need to be verified response, err := qev.Verify("EMAIL") if err != nil { panic(err) } print(response.Result)
import java.io.BufferedReader; import java.io.DataOutputStream; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; import javax.net.ssl.HttpsURLConnection; public class QevSingleAPI { public static void main(String[] args) throws Exception { QevSingleAPI http = new QevSingleAPI(); http.sendGet(); } // HTTP GET request private void sendGet() throws Exception { // Replace API_KEY with your API Key String API_KEY = "API_KEY"; // Email address which need to be verified String EMAIL = "EMAIL"; String url = "https://api.quickemailverification.com/v1/verify?email="+EMAIL+"&apikey="+API_KEY; URL obj = new URL(url); HttpURLConnection con = (HttpURLConnection) obj.openConnection(); // optional default is GET con.setRequestMethod("GET"); int responseCode = con.getResponseCode(); System.out.println("\nSending 'GET' request to URL : " + url); System.out.println("Response Code : " + responseCode); BufferedReader in = new BufferedReader( new InputStreamReader(con.getInputStream())); String inputLine; StringBuffer response = new StringBuffer(); while ((inputLine = in.readLine()) != null) { response.append(inputLine); } in.close(); //print result System.out.println(response.toString()); } }
using System; using System.Web; using System.Net; using System.IO; namespace QevSingleAPI { class QevSingleAPI { static void Main() { try { // Replace API_KEY with your API Key string apiKey = "API_KEY"; // Email address which need to be verified string emailToValidate = "EMAIL"; string responseString = ""; string apiURL = "https://api.quickemailverification.com/v1/verify?email=" + HttpUtility.UrlEncode(emailToValidate) + "&apikey=" + apiKey; HttpWebRequest request = (HttpWebRequest)WebRequest.Create(apiURL); request.Method = "GET"; using (WebResponse response = request.GetResponse()) { using (StreamReader ostream = new StreamReader(response.GetResponseStream())) { responseString = ostream.ReadToEnd(); } } } catch (Exception ex) { //Catch Exception - All errors will be shown here - if there are issues with the API } } } }
Imports System.Net Imports System.IO Module QevSingleAPI Sub Main() Try ' Replace API_KEY with your API Key Dim apiKey as string = "API_KEY" ' Email address which need to be verified Dim emailToVerify as string = "EMAIL" Dim responseString as string = "" Dim apiURL as string = "https://api.quickemailverification.com/v1/verify?email=" & System.Web.HttpUtility.UrlEncode(emailToVerify) & "&apikey=" & apiKey Dim request As HttpWebRequest = DirectCast(WebRequest.Create(apiURL), HttpWebRequest) request.Method = "GET" Using response As WebResponse = request.GetResponse() Using ostream As New StreamReader(response.GetResponseStream()) responseString = ostream.ReadToEnd() Console.WriteLine(responseString) End Using End Using Catch ex as exception ' Catch Exception - All errors will be shown here - if there are issues with the API Console.WriteLine(ex) End Try End Sub End Module
using System; using System.Net.Http; using System.Net.Http.Json; using System.Text.Json; using System.Threading.Tasks; using System.Web; namespace QevSingleAPI { class Validate { // Replace API_KEY with your API Key private const string QuickVerificationEmailApiKey = "API_KEY"; static async Task Main(string[] args) { // Email address which need to be verified string emailAddress = "EMAIL"; EmailVerificationModel model = await ValidateEmailAddressAsync(emailAddress); Console.WriteLine(emailAddress); Console.WriteLine(model.ToString()); Console.ReadLine(); } public static async Task<EmailVerificationModel> ValidateEmailAddressAsync(string emailAddress) { try { using (HttpClient httpClient = new HttpClient()) { string apiURL = $"https://api.quickemailverification.com/v1/verify?email={HttpUtility.UrlEncode(emailAddress)}&apikey={QuickVerificationEmailApiKey}"; return await httpClient.GetFromJsonAsync<EmailVerificationModel>(apiURL); } } catch (Exception) { throw; } } } [Serializable] public class EmailVerificationModel { public string result { get; set; } public string reason { get; set; } public string disposable { get; set; } public string accept_all { get; set; } public string role { get; set; } public string free { get; set; } public string email { get; set; } public string user { get; set; } public string domain { get; set; } public string mx_record { get; set; } public string mx_domain { get; set; } public string safe_to_send { get; set; } public string did_you_mean { get; set; } public string success { get; set; } public object message { get; set; } public EmailVerificationModel() { } public override string ToString() { return this.Serialize(); } } public static class StringExtensions { public static string Serialize(this object dataToSerialize) { if (dataToSerialize == null) return null; var options = new JsonSerializerOptions { WriteIndented = true }; return JsonSerializer.Serialize(dataToSerialize, options); } } }
When you have list of emails to be verified, it can be verified either by uploading list from client area, or use this API to upload and verify email list. All you need to verify email list over API is an API key and a list of emails to be verified.
curl -X POST -F 'upload=@/home/user/email_list.csv' \ 'https://api.quickemailverification.com/v1/bulk-verify' \ -H 'Authorization:token API_KEY' \ -H 'X-QEV-Filename:email_list.csv' \ -H 'X-QEV-Callback:http://www.yoursite.com/qev-callback'
<?php $headers = array(); // Replace API_KEY with your API Key $headers[] = "Authorization:token API_KEY"; // Set the display name of file to upload (optional) $headers[] = "X-QEV-Filename:email_list.csv"; // Set your callback URL (optional) $headers[] = 'X-QEV-Callback:http://www.yoursite.com/qev-callback'; // This is a path to your file to upload $post = array('upload' => '@/home/user/email_list.csv'); $ch = curl_init("https://api.quickemailverification.com/v1/bulk-verify"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, $post); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 15); curl_setopt($ch, CURLOPT_TIMEOUT, 30); $result = curl_exec($ch); $jsonToArray = json_decode($result, true); curl_close($ch); ?>
var fs = require('fs'); var request = require('request'); request.post({ url: 'https://api.quickemailverification.com/v1/bulk-verify', headers: {'Authorization':'token API_KEY', // Replace API_KEY with your API Key 'X-QEV-Filename':'email_list.csv', // Set the display name of file to upload (optional) 'X-QEV-Callback':'http://www.yoursite.com/qev-callback' // Set your callback URL (optional) }, formData: { file: fs.createReadStream('/home/user/email_list.csv'), } }, function(error, response, body) { console.log(body); });
import requests import mimetypes file_to_upload = '/home/user/email_list.csv' file_mime = mimetypes.guess_type(file_to_upload)[0] # Returns mime_type of file to upload headers = { 'Authorization': 'API_KEY', # Replace API_KEY with your API Key 'X-QEV-Filename': 'email_list.csv', # Set the display name of file to upload (optional) 'X-QEV-Callback': 'http://www.yoursite.com/qev-callback' # Set your callback URL (optional) } files = { 'upload': (file_to_upload, open(file_to_upload, 'r'), file_mime) } response = requests.post('https://api.quickemailverification.com/v1/bulk-verify', headers=headers, files=files, timeout=(15, 30)) qevResponse = response.json() print(qevResponse)
require 'uri' require 'net/http' require 'net/http/post/multipart' # Returns mime_type of file to upload mime_type = `file --b --mime-type '/home/user/email_list.csv'`.strip url = URI.parse('https://api.quickemailverification.com/v1/bulk-verify') request = Net::HTTP::Post::Multipart.new url.path, "file" => UploadIO.new('/home/user/email_list.csv', mime_type) # Replace API_KEY with your API Key request.add_field("Authorization", "token API_KEY") # Set the display name of file to upload (optional) request.add_field("X-Qev-Filename", "email_list.csv") # Set your callback URL (optional) request.add_field("X-Qev-Callback", "http://www.yoursite.com/qev-callback") res = Net::HTTP.start(url.host, url.port) do |http| response = http.request(request) puts response.body end
package main import ( "bytes" "fmt" "io" "log" "mime/multipart" "net/http" "os" "path/filepath" ) func main() { request, err := setFileUpload("https://api.quickemailverification.com/v1/bulk-verify", "upload", "/home/user/email_list.csv") // Replace API_KEY with your API Key request.Header.Set("Authorization", "token API_KEY") // Set the display name of file to upload (optional) request.Header.Set("X-QEV-Filename", "email_list.csv") // Set your callback URL (optional) request.Header.Set("X-QEV-Callback", "http://www.yoursite.com/qev-callback") client := &http.Client{} resp, err := client.Do(request) if err != nil { log.Fatal(err) } else { body := &bytes.Buffer{} _, err := body.ReadFrom(resp.Body) if err != nil { log.Fatal(err) } resp.Body.Close() fmt.Println(body) } } func setFileUpload(uri string, paramName, path string) (*http.Request, error) { file, err := os.Open(path) if err != nil { return nil, err } defer file.Close() body := &bytes.Buffer{} writer := multipart.NewWriter(body) part, err := writer.CreateFormFile(paramName, filepath.Base(path)) if err != nil { return nil, err } _, err = io.Copy(part, file) err = writer.Close() if err != nil { return nil, err } req, err := http.NewRequest("POST", uri, body) req.Header.Set("Content-Type", writer.FormDataContentType()) return req, err }
using System; using System.Net.Http; using System.IO; using System.Threading.Tasks; namespace QevListAPI { class QevListAPI { static void Main() { try { using (var client = new HttpClient()) { // Replace API_KEY with your API Key client.DefaultRequestHeaders.Add("Authorization", "token API_KEY"); // Set the display name of file to upload (optional) client.DefaultRequestHeaders.Add("X-Qev-Filename", "email_list.csv"); // Set your callback URL (optional) client.DefaultRequestHeaders.Add("X-Qev-Callback", "http://www.yoursite.com/qev-callback"); using (var content = new MultipartFormDataContent()) { var path = @"/home/user/email_list.csv"; FileStream fs = File.OpenRead(path); var streamContent = new StreamContent(fs); streamContent.Headers.Add("Content-Type", "application/octet-stream"); content.Add(streamContent, "file", Path.GetFileName(path)); Task<HttpResponseMessage> message = client.PostAsync("https://api.quickemailverification.com/v1/bulk-verify", content); var Response = message.Result.Content.ReadAsStringAsync(); Console.WriteLine(Response.Result); } } } catch (Exception ex) { //Catch Exception - All errors will be shown here - if there are issues with the API } } } }